Running Atmel Studio 4 on Win10
Back to Ian M's profile
This is *NOT* a tutorial on coding in AVR assembly language. Far better resources for that than anything I can write exist on the WWW. If you've got a new fast PC, with lots of RAM, and a currently supported AVR programmer/debugger you may be better off ignoring this whole page and installing Microchip's current IDE!
Resurrecting an Atmel STK500
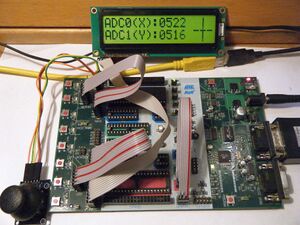
Thanks to another member, I recently was given a 2006 vintage Atmel STK500 AVR development board/programmer (originally released in early 2001), with a few AVR MCUs thrown in as well. IMHO its worth resurrecting the old STK500 even though I've got a choice of more modern USB AVR programmers, as its one of the few programmers that can do 'fuse-busting' i.e. HV serial programming with an external clock, or even HV parallel programming, to recover an Atmel AVR family MCU that you've been dumb enough to set fuses for that it cant run with!
Unfortunately it didn't come with any cables, and as I didn't have any IDC connectors in the right sizes, I spent an evening crimping about 70 female duPont contacts to make up 6" replacements for the 2x 10 way and 1x 6 way IDC cables and 4x 2way jumper cables it originally was sold with (See TME STK500 page). I'm using a Prolific PL2303 (clone) USB<=>Serial adaptor rather than a DE9 M-F cable as originally supplied, as I don't currently have a working legacy COM port to plug it into!
The Prolific clone serial adapter has a few driver issues under Win10 - see: http://wp.brodzinski.net/2014/10/01/fake-pl2303-how-to-install/
I am also interested in learning AVR assembly language properly as up to now, I've only programmed AVRs in C, and if you don't have a good understanding of what is (or is likely to be) happening 'under the hood', you have very little idea when you are asking the MCU to do something unreasonably complex, and run into unexpected performance problems.
N.B. The original sample assembler code from the STK500 user guide "Example Application: Using LEDs and Switches" is for the obsolete and discontinued AT90S8515. Use an ATmega8515 with the 'S8515C' compatibility fuse set, or change the .include line's *def.inc file for the ATmega8515 without the compatibility fuse (m8515def.inc) as has been done here, or similarly for just about any other 40 pin PDIP ATmega. Also, it does *NOTHING* until you press a button, and some of the buttons have no visible effect if none of the LEDs are lit. Hold SW1 and the LEDs should count up in binary.
Installing Atmel AVR Studio 4 on Win10 x64
The current development environment 'Microchip Studio for AVR and SAM Devices' (a slightly up-versioned and rebranded Atmel Studio 7) is notorious for being bloatware and running very sluggishly on older PCs, and the general consensus is that for assembler projects using older AVR MCUs and hardware tools the best option is Atmel AVR Studio 4, which is still downloadable from the Microchip AVR archives (scroll down to: AVR® Studio 4.18). The final release v4.19.730 is known to be borked - the toolchains have to be entered manually for every new project - so its best to install AVR Studio v4.18.684, and upgrade it to v4.18.716 using the AVR Studio v4.18.SP3.716 service pack installer.
Unfortunately v4.18.684 is signed with an expired Atmel certificate, so wont install on Win 10 x64 OSes. The easy fix is to un-sign the downloaded installer (e.g. use the free Fluxbytes Fileunsigner utility). It can then be installed successfully on Win10 x64 provided you do *NOT* let it install the Jungo USB drivers (required for Atmel hardware tools with a USB interface). Fortunately the v4.18.SP3.716 service pack installer still has a valid certificate, so *CAN* install the Jungo drivers - if you need them.
Installing the WINAVR C compiler for use in AVR Studio 4
If you want to program and debug AVR MCUs in C under AVR Studio 4, you'll need an AVR C cross-compiler. WINAVR provides an AVR-GCC toolchain, compatible with AVR Studio 4, but hasn't been maintained in a *very* long time. Get WINAVR from https://sourceforge.net/projects/winavr/files/WinAVR/20100110/ and install it in its default location (C:\WinAVR-20100110).
- CAUTION: there have been some reports of it trashing people's PATH system variable, so you may wish to backup your PATH to a text file before running the installer. To do so, open Settings (the gear icon in the Win10 start menu or action center) and paste advanced system settings into the 'Find a setting' search box, then click 'View advanced system settings' which appears immediately above the search box. Next click the 'Environment Variables...' button and it will open an Environment Variables dialog, with two sections, your user and system variables. Parts of your PATH probably exist in both - the system PATH is the most important but if you have any applications installed for only your user, you've probably got a user path as well. To copy the path from each section you need to select it, click the 'Edit...' button then click the 'Edit text...' button. Copy the variable value, paste it into your backup text file, with a note on the line above whether it was the system path or the user one, then Cancel out of the dialog so *NOTHING* is changed.
AVR Studio 4 under Win10 x64 (and other 64 bit versions of Windows) has problems building with WINAVR due to a bug in the CreateProcess API function used by MSYS/MingW (platform for some of the utilities in WinAVR). See https://www.madwizard.org/electronics/articles/winavrvista for details. The fix is to download msys-1.0-vista64.zip from that page, and replace C:\WinAVR-20100110\utils\bin\msys-1.0.dll with the one from the zip file.
Here's a minimal C LED blinky 'Hello world' that runs on the STK500 demo board:
//For a 40 pin AVR on a STK500 board #define F_CPU 3686400 #include <avr/io.h> #include <util/delay.h> int main() { DDRB |= (1 << DDB7); // Make PB7 (Port B header pin 8) be an output. while(1) { PORTB |= (1 << PORTB7); // Turn LED7 on. _delay_ms(500); PORTB &= ~(1 << PORTB7); // Turn LED7 off. _delay_ms(500); } }
AVR Studio 4 Quirks
AVR Studio 4.18 does have a few quirks you need to be aware of:
- It tends to crash if you close one project and open another - its best to close and reopen the whole application after closing a project.
- If you close the simulator/debugger 'I/O View' pane, you wont be able to find it again in any of the menus or toolbars! To get it back, either right click the status bar, (right at the bottom of the main window) and select it or press: Alt+5
- The Programmer utility (used for STK500 and various others) does NOT pick up the location of the HEX file to be programmed from the project properties. Whenever you change projects, always check its using the correct HEX file or it will grab one from a previous project and you'll be wondering why your new code changes have no effect!
- If you have problems programming an AVR, check it has a fast enough clock for the current programming frequency! Sometimes the programmer utility and/or STK500 seem to forget their target clock settings. If the 'Read Signature' button in the 'Main' tab of the programmer window doesn't read the correct signature, and you've checked everything is properly connected and the AVR powered, the programming clock speed is probably the issue. Some AVRs default to running from a slow internal oscillator after being erased, so you may have to lower the ISP frequency (click 'Settings...' on that tab) to program the fuses to use a faster clock source. The STK500's Clock Generator output is set on the 'HW settings' tab of the programmer utility.
- Once the programmer utility is connected, correctly configured and you've checked the chip's signature, you can minimise the utility and do 'one click' programming using the toolbar buttons in the main application window.
- The simulator/debugger 'Watch' window is rather limited - I still haven't figured out how to watch a register pair as a single entity (or even if that's possible), and am having to watch the registers in the pair individually. Hilite a register name (or alias) or variable name in your source and drag it into the watch window to watch that register or variable.
Other useful tools and resources
Gerhard Schmidt's AVR Assembler 'boilerplate' generator: http://www.avr-asm-tutorial.net/avr_en/avr_head/avr_head.html takes much of the drudgery out of setting up the main Assembler source file for a new project. I can also highly recommend the other resources at http://www.avr-asm-tutorial.net/
Atmel's old AVRfreaks forum (historically the main community for non-Arduino AVR programming) is now behind a Microchip registration wall. You need to register at Microchip.com to even view it! :(
Microchip's registration page seemed to be half-way borked and I never received the confirmation email required to properly set up a Microchip account, but whatever it did seems to be good enough to let me into https://www.avrfreaks.net/ to view the forums. Unfortunately Microchip have borked AVRfreaks, and most incoming Google links to topics there will take you to a landing page that may offer you several topics to choose from. When you get to a topic it seems that any attachments have been lost, though maybe I just need to register with AVRfreaks to see them (if my half-borked Microchip registration will let me).